Nougat Documentation
II - Nougat as Language
2.1 Influences
Programming languages such as C, Alef++, JavaScript, Perl, Go, Python, and others are languages that has some influence in Nougat, specially Go with its go statement, JavaScript and Perl with their dynamism in scripting, and C in primal syntatic structure. As for instance, JavaScript declaration for a function would look a lot like this:
funtion add(x, y) {
return x + y;
}
In Nougat, the function declaration above will be translated as:
fun add(x, y) {
return x + y;
}
This makes the Nougat programming langauge easier to learn for those who had a little experience in coding despite of its' syntax complixity.
2.2 Backus-Naur Form Definition
According to Wikipedia, Backus-Naur Form is a metasyntax notation for context-free grammars, often used to describe the syntax of languages used in computing, such as computer programming languages, document formats, instruction sets and communication protocols.
2.2.1 Reserved Keywords
The below list of keywords are the so-called "reserved keywords," which are words that cannot be used as identifier(s) in Nougat.
break case catch continue default
defer do else false finally
fun go if include iter
nil of render return switch
throw try true while var
2.2.2 Grammar Definition
Here is the complete grammar definition of Nougat's syntax and language rules.
Nougat := (IncludeStmt | VarDeclStmt | FuncDeclStmt)*
IncludeStmt :=
"include" <STRING> ("," <STRING>)* ";"
VarDeclStmt :=
"var" <IDENTIFIER> ["=" Expression]
("," <IDENTIFIER> ["=" Expression])* ";"
FuncDeclStmt :=
"fun" <IDENTIFIER>
"(" [<IDENTIFIER> ["=" Expression] ("," <IDENTIFIER> ["=" Expression])*] ")"
Statement
Statement :=
BlockStmt | VarDeclStmt | DeferStmt | RenderStmt |
IfStmt | WhileStmt | DoWhileStmt | ReturnStmt |
ThrowStmt | GoStmt | SwitchStmt | TryCatchFinallyStmt |
IterStmt | BreakStmt | ContinueStmt | FuncDeclStmt |
ExpressionStmt
BlockStmt := (Statement)*
DeferStmt := "defer" Statement
RenderStmt := "render" Expression ";"
IfStmt :=
"if" "(" Expression ")" Statement
["else" Statement]
WhileStmt := "while" "(" Expression ")" Statement
DoWhileStmt :=
"do" Statement
"while" "(" Expression ")" ";"
ReturnStmt := "return" Expression ";"
ThrowStmt := "throw" Expression ";"
GoStmt := "go" Statement
SwitchStmt :=
"switch" "(" Expression ")"
"{"
(("case" Expression ":")* Statement)*
["default" ":" Statement]
"}"
TryCatchFinallyStmt :=
"try" Statement
"catch" ["(" <IDENTIFIER> ")"] Statement
["finally" Statement]
IterStmt :=
"iter" "(" <IDENTIFIER> "of" <IDENTIFIER> ")"
Statement
BreakStmt := "break" ";"
ContinueStmt := "continue" ";"
ExpressionStmt := Expression ("," Expression)* ";"
Expression := LogicExpr
LogicExpr := NilCoalescingExpr (("&&" | "||") NilCoalescingExpr)*
NilCoalescingExpr := EqualityExpr ("?" EqualityExpr)*
EqualityExpr := ComparisonExpr (("==" | "!=" | "=") ComparisonExpr)*
ComparisonExpr := TermExpr (("<" | ">" | "<=" | ">=") TermExpr)*
TermExpr := FactorExpr (("+" | "-") FactorExpr)*
FactorExpr := UnaryExpr (("/" | "*" | "%") UnaryExpr)*
UnaryExpr := (["!" | "+" | "-" | "~"] Expression) | PrimaryExpr
ObjectInitExpr :=
"&" ("[" [Expression ("," Expression)*] "]" |
"{" [<IDENTIFIER> [":" Expression] ("," <IDENTIFIER> [":" Expression])*] "}")
GroupedExpr := "(" Expression ")"
LambdaExpr :=
"fun" <IDENTIFIER>
"(" [<IDENTIFIER> ["=" Expression] ("," <IDENTIFIER> ["=" Expression])*] ")"
Statement
PrimaryExpr :=
(<STRING> | <DIGIT> | LambdaExpr |
<IDENTIFIER> ("." <IDENTIFIER>)* |
"true" | "false" | "nil" |
ObjectInitExpr | GroupedExpr)
("[" Expression "]" | "(" [Expression ("," Expression)*] ")")*
2.3 Execution Procedures
Nougat is a just-in-time compiled language, thus, source codes are compiled and executed at runtime. Being said, it follows an execution procedure as shown in the diagram below:
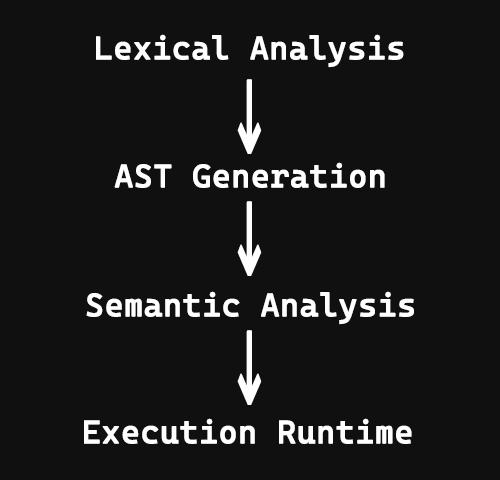
The Nougat JIT process starts with lexical analysis phase and ends with exeuction/runtime. However, each phase can be halted if any error occured.
2.3.1 Lexical Analysis
The lexical analysis phase is the process whereare the input source code are being scanned and is identified. Hence, it'll be converted into token that contains informations such as source file, location (line and column), token type, and of course it's own image.
2.3.2 Abstract Syntax Tree
Abstract Syntax Tree is a representation of linkage and structure of a source code, hence, this phase is where the tokens from lexical analysis are being analyzed base on the grammatical rules of Nougat. However, if an error has encountered the parsing phase might be halted. Additionally, this phase generates informations that can be resolved and visited later on the semantic analysis and execution phase.
2.3.3 Semantic Analysis
After the generation ASTs (Abstract Syntax Trees), datas being held are now available to be walked in via linkage generated within each AST. Whereas, symbols, names, and/or identifiers are being resolve. In this phase, already used or undeclared names/identifiers are reported, if then, the compilation is halted.
2.3.4 Execution/Runtime
The final phase of compilation is the execution itself. In this phase, after the successful semantic analysis, Nougat then proceeds to execute each AST-generated details, on the other hand, this is where the source code gets executed.